Arduino is a versatile platform that has revolutionized the world of DIY electronics and prototyping. From simple LED blinkers to complex robotic systems, Arduino projects come in all shapes and sizes. However, as the complexity of your project grows, managing different states and behaviors can become a daunting task. This is where state machines come to the rescue. In this blog post, we’ll explore how to leverage the power of state machines to create well-structured, efficient, and scalable Arduino projects.
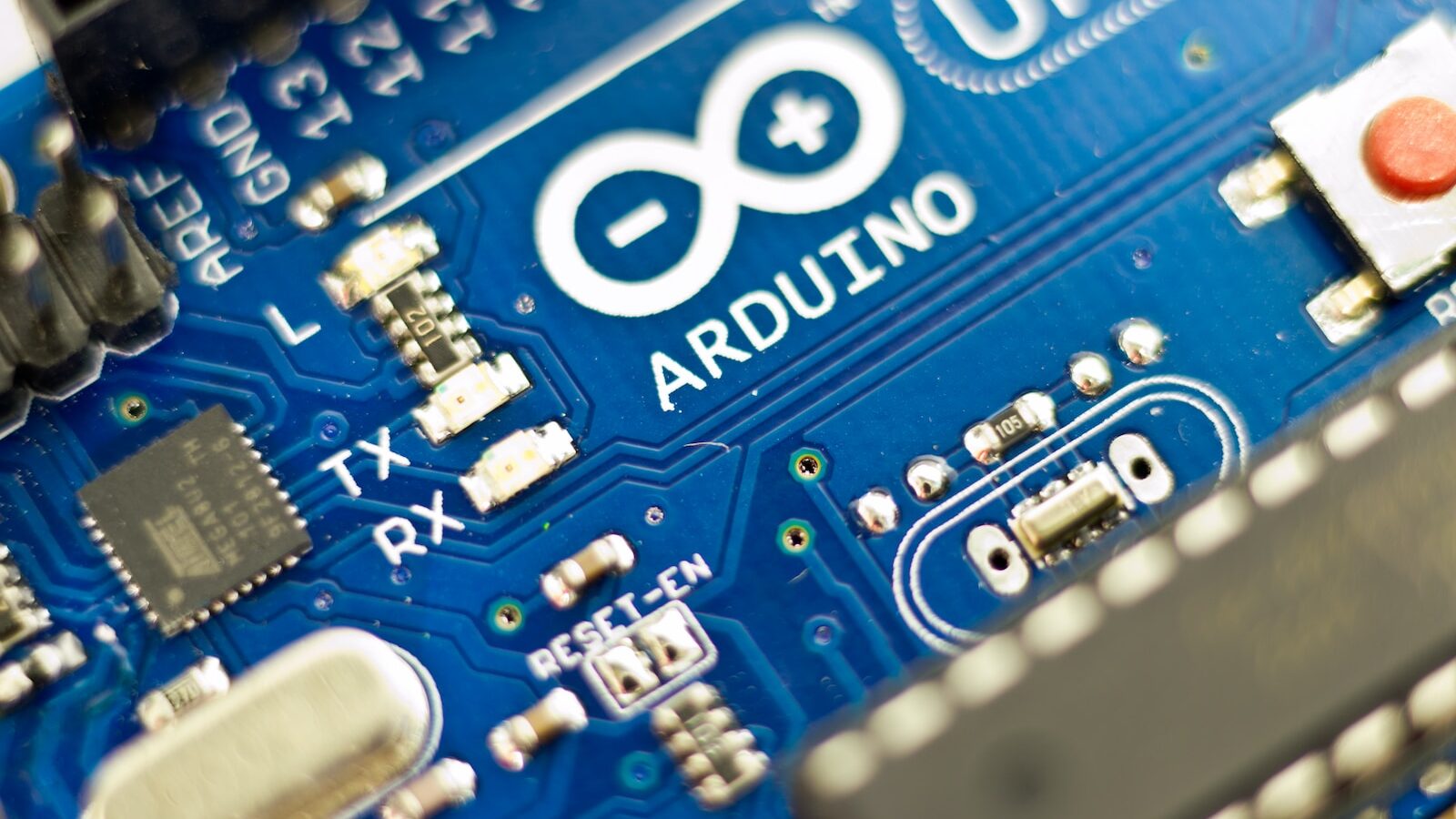
State Machine in Arduino
Why Use State Machines?
State machines provide a systematic approach to handling complex tasks by breaking them down into distinct states and transitions. By organizing your code in this way, you can achieve several benefits:
Clearer Code Structure: State machines promote modular code organization, making it easier to understand, debug, and maintain your project. Each state represents a specific behavior or condition, allowing you to focus on specific tasks within that state.
Concurrent Task Handling: With a state machine, you can handle multiple tasks concurrently without blocking or delaying critical operations. By dividing your project into states, you can prioritize and manage different tasks independently.
Flexibility and Scalability: State machines offer a flexible framework that facilitates future modifications and extensions to your project. Adding new states or transitions becomes more straightforward, making your project adaptable to changing requirements.
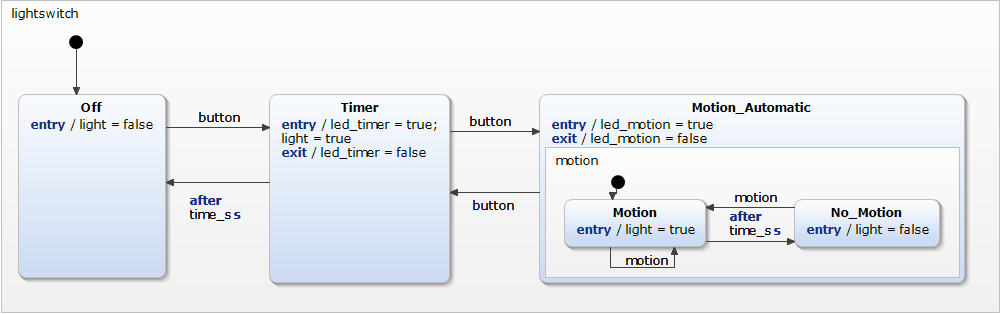
Implementing a State Machine in Arduino:
Let’s dive into a step-by-step approach for implementing a state machine in your Arduino project:
Step 1: Identify the States: Begin by identifying the different states your system can be in. Consider the various behaviors, conditions, or modes that your project needs to handle.
Step 2: Define Transitions: Determine the events or conditions that trigger transitions from one state to another. These could be user inputs, sensor readings, time-based events, or any other criteria specific to your project.
Step 3: Code Implementation: In your Arduino sketch, define an enum or constants to represent the states. Create a variable to track the current state of your system. Use control structures like switch-case or if-else statements to handle transitions based on the current state and trigger events.
Step 4: Write Code for Each State: Within each state, write the necessary code to perform the desired actions or tasks. This can include reading sensors, controlling actuators, making decisions based on inputs, or any other logic relevant to that state.
Step 5: Update the State: As your program executes, update the state variable based on the defined transitions. This ensures that your system moves from one state to another when the appropriate conditions are met.
Here’s a basic example of an Arduino code implementing a state machine:
// Define the states
enum State {
STATE_IDLE,
STATE_LED_ON,
STATE_LED_OFF
};
// Define the variables
int ledPin = 13;
State currentState = STATE_IDLE;
// Setup function runs once at the beginning
void setup() {
pinMode(ledPin, OUTPUT);
}
// Loop function runs repeatedly
void loop() {
// State machine logic
switch (currentState) {
case STATE_IDLE:
// Code for the idle state
// For example, wait for a button press to change the state
if (digitalRead(buttonPin) == HIGH) {
currentState = STATE_LED_ON;
}
break;
case STATE_LED_ON:
// Code for the LED on state
digitalWrite(ledPin, HIGH);
delay(1000); // LED on delay
currentState = STATE_LED_OFF;
break;
case STATE_LED_OFF:
// Code for the LED off state
digitalWrite(ledPin, LOW);
delay(1000); // LED off delay
currentState = STATE_IDLE;
break;
}
}
Code language: C++ (cpp)
In this example, we have three states: STATE_IDLE
, STATE_LED_ON
, and STATE_LED_OFF
. The program starts in the STATE_IDLE
state, waiting for a button press. Once the button is pressed, it transitions to the STATE_LED_ON
state, turns on an LED for one second, and then transitions to the STATE_LED_OFF
state, turning off the LED for one second. Finally, it transitions back to the STATE_IDLE
state, waiting for another button press to repeat the cycle.
Note that this is a simplified example, and in a practical scenario, you would likely have additional conditions and transitions based on your project’s requirements.
Here’s another example of an Arduino code implementing a state machine with more advanced features:
// Define the states
enum State {
STATE_IDLE,
STATE_COUNTDOWN,
STATE_ALARM,
STATE_RESET
};
// Define the variables
int buttonPin = 2;
int ledPin = 13;
int count = 10;
State currentState = STATE_IDLE;
// Setup function runs once at the beginning
void setup() {
pinMode(buttonPin, INPUT_PULLUP);
pinMode(ledPin, OUTPUT);
Serial.begin(9600);
}
// Loop function runs repeatedly
void loop() {
// State machine logic
switch (currentState) {
case STATE_IDLE:
// Code for the idle state
Serial.println("Press the button to start the countdown");
digitalWrite(ledPin, LOW);
if (digitalRead(buttonPin) == LOW) {
currentState = STATE_COUNTDOWN;
}
break;
case STATE_COUNTDOWN:
// Code for the countdown state
Serial.print("Countdown: ");
Serial.println(count);
digitalWrite(ledPin, HIGH);
delay(1000);
count--;
if (count <= 0) {
currentState = STATE_ALARM;
}
break;
case STATE_ALARM:
// Code for the alarm state
Serial.println("Time's up!");
digitalWrite(ledPin, LOW);
delay(200);
digitalWrite(ledPin, HIGH);
delay(200);
currentState = STATE_RESET;
break;
case STATE_RESET:
// Code for the reset state
Serial.println("Resetting...");
count = 10;
delay(1000);
currentState = STATE_IDLE;
break;
}
}
Code language: C++ (cpp)
In this example, we have four states: STATE_IDLE
, STATE_COUNTDOWN
, STATE_ALARM
, and STATE_RESET
. The program starts in the STATE_IDLE
state, waiting for a button press to start the countdown. Once the countdown starts in the STATE_COUNTDOWN
state, the count value decrements every second. When the count reaches zero, it transitions to the STATE_ALARM
state, where the LED blinks rapidly to indicate that time is up. After that, it transitions to the STATE_RESET
state, where the count is reset to 10 and a delay of one second occurs before transitioning back to the STATE_IDLE
state.
This example demonstrates a more complex state machine with additional features like countdown functionality, user input, LED control, and serial communication for debugging purposes. You can modify and expand upon this example to suit your specific project requirements.
Real-World Arduino Projects That Benefit from State Machines
Home Automation System:
A home automation system typically involves controlling various devices like lights, fans, and appliances based on user inputs or sensor readings. By implementing a state machine, you can manage different states such as “Idle,” “Lights On,” “Fan On,” “Appliance Control,” etc. Transitions can be triggered by user commands or sensor inputs, allowing for a well-organized and responsive home automation system.
Temperature and Humidity Control:
In projects that involve monitoring and controlling temperature and humidity, a state machine can be used to handle different states like “Monitoring,” “Heating,” “Cooling,” and “Maintaining.” Transitions can be based on readings from temperature and humidity sensors, ensuring precise control and efficient energy usage.
Traffic Light Control System:
Traffic light control systems require managing the states of multiple traffic signals. By implementing a state machine, you can define states such as “Green Light,” “Yellow Light,” and “Red Light” for each signal. Transitions can be triggered by timers or by detecting vehicle presence using sensors, allowing for synchronized and efficient traffic flow.
Robotic Arm Control:
Robotic arms often require precise control and coordination of different movements. By using a state machine, you can define states like “Idle,” “Moving Up,” “Moving Down,” “Rotating Left,” “Rotating Right,” etc. Transitions can be triggered by user commands or feedback from position sensors, enabling smooth and accurate robotic arm control.
Security System:
A security system may have states such as “Armed,” “Disarmed,” “Alarm Triggered,” and “Alert Mode.” Transitions can be triggered by user inputs, motion sensors, or door/window sensors. By using a state machine, you can create a robust security system that handles different scenarios efficiently and provides appropriate responses.
These are just a few examples, and the possibilities are endless. State machines can be applied to a wide range of Arduino projects, providing structure, modularity, and flexibility. They help in managing complex behaviors, coordinating multiple components, and creating responsive systems that are easy to understand and maintain.
Ways State Machines Benefit Complex Behavior Management in Arduino Projects
Modular Code Organization:
State machines promote modular code organization by dividing the project into distinct states. Each state represents a specific behavior or condition, allowing you to focus on individual tasks within that state. This modular approach makes the code more structured, easier to understand, and maintainable.
Clear State Transitions:
By defining transitions between states, state machines provide a clear and explicit way to manage complex behaviors. Transitions can be triggered by various events, such as user inputs, sensor readings, or time-based conditions. This makes it easier to control the flow of the program and handle different scenarios effectively.
Concurrent Task Handling:
One of the challenges in complex Arduino projects is managing multiple tasks concurrently. State machines allow you to handle different tasks independently within each state. This means you can perform simultaneous actions, such as reading sensors, controlling actuators, or processing inputs, without blocking or delaying critical operations.
Responsive and Adaptive Systems:
State machines enable you to create responsive and adaptive systems. Depending on the current state and triggered events, the system can dynamically adjust its behavior and respond accordingly. This flexibility is crucial in projects where conditions can change rapidly, allowing your Arduino project to adapt and provide appropriate responses in real-time.
Scalability and Extensibility:
As the complexity of your project grows or new requirements arise, state machines offer scalability and extensibility. Adding new states or transitions becomes more manageable, allowing you to expand the project’s functionality without drastically modifying the existing code. This flexibility makes it easier to accommodate future enhancements or modifications to your Arduino project.
By using state machines, you can effectively manage complex behaviors, coordinate different tasks, and create well-structured, efficient, and scalable Arduino projects. Whether it’s managing multiple sensors, controlling actuators, or handling diverse inputs, state machines provide a systematic approach that enhances the overall functionality and maintainability of your project.
State Machine Libraries
There are several libraries and resources available that can assist in implementing state machines in Arduino projects. Here are a few popular ones:
- Arduino State Machine Library (Arduino-SM):
Arduino-SM is a lightweight library specifically designed for implementing state machines in Arduino projects. It provides an intuitive syntax and offers features like automatic transition handling, event-based state changes, and state entry/exit actions. The library is easy to use and comes with comprehensive documentation and examples to get you started quickly. - Finite State Machine (FSM) Arduino Library:
The FSM Arduino Library is another widely used library for implementing state machines. It offers a simple and flexible API for creating and managing state machines in Arduino projects. The library supports defining states, transitions, and actions, and provides methods to handle events and perform state transitions. - PlatformIO State Machine Library:
If you’re using the PlatformIO development platform for Arduino, the PlatformIO State Machine Library is a great choice. It provides a set of features for creating state machines, including state transitions, event handling, and action execution. The library integrates well with the PlatformIO ecosystem and offers clear documentation and examples. - Tinkr State Machine:
Tinkr State Machine is a lightweight state machine library specifically designed for resource-constrained Arduino projects. It provides a minimalistic approach to state machine implementation and focuses on simplicity and efficiency. It offers state transitions, event handling, and basic action execution.
Additionally, there are numerous online resources and tutorials available that can help you understand and implement state machines in Arduino projects. Websites like Arduino.cc, Adafruit, and GitHub have extensive documentation, examples, and community forums where you can find valuable insights and guidance on implementing state machines.
Remember to choose a library or resource that best suits your project’s requirements and aligns with your coding preferences. Reading through the documentation and examples provided by these libraries will help you get started quickly and efficiently implement state machines in your Arduino projects.
Final Thoughts
In conclusion, state machines have proven to be invaluable in managing complex behaviors within Arduino projects. By employing state machines, developers can achieve a modular code organization, enabling clearer structure and easier maintenance. The ability to handle concurrent tasks and prioritize actions enhances the efficiency and responsiveness of Arduino projects. State machines also provide the flexibility to adapt and scale projects as requirements evolve, ensuring long-term viability.
Whether it’s controlling home automation systems, managing temperature and humidity, coordinating traffic lights, controlling robotic arms, or implementing security systems, state machines offer a systematic approach to handle diverse behaviors. They provide a clear framework for defining states, transitions, and actions, enabling seamless control and coordination of project components.
By embracing state machines, Arduino enthusiasts can unlock the full potential of their projects. The well-organized structure, efficient task handling, and adaptability of state machines create a solid foundation for building robust and scalable Arduino applications. Embrace the power of state machines and elevate your Arduino projects to new heights of efficiency, maintainability, and functionality.
So, next time you embark on an Arduino project, consider integrating state machines into your design. Leverage their benefits to streamline complex behaviors, optimize performance, and create innovative and responsive systems that captivate and inspire. Happy coding and exploring the possibilities of state machines in your Arduino journey!